DOM Manipulation In TypeScript
Learn DOM Manipulation In TypeScript
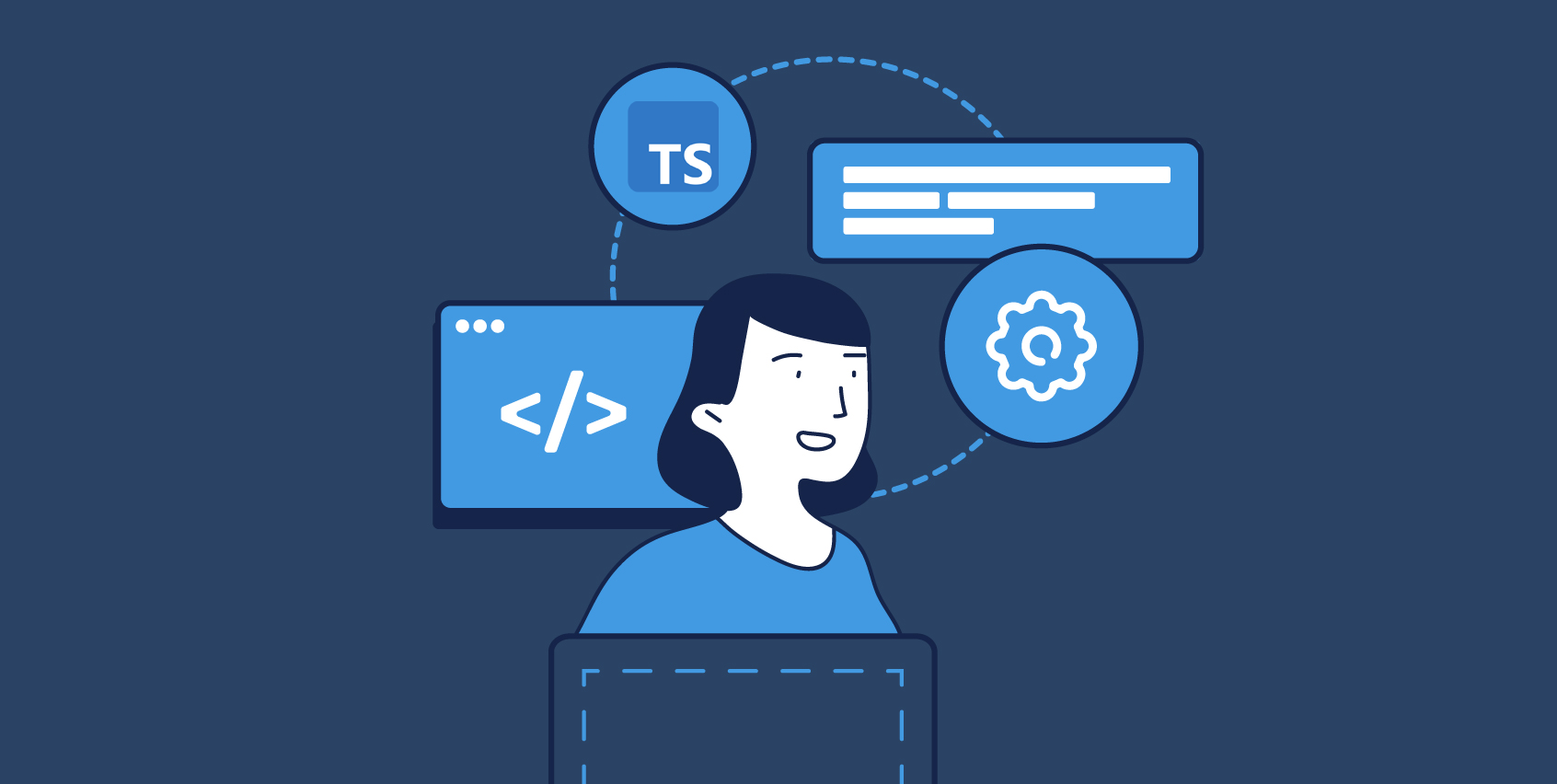
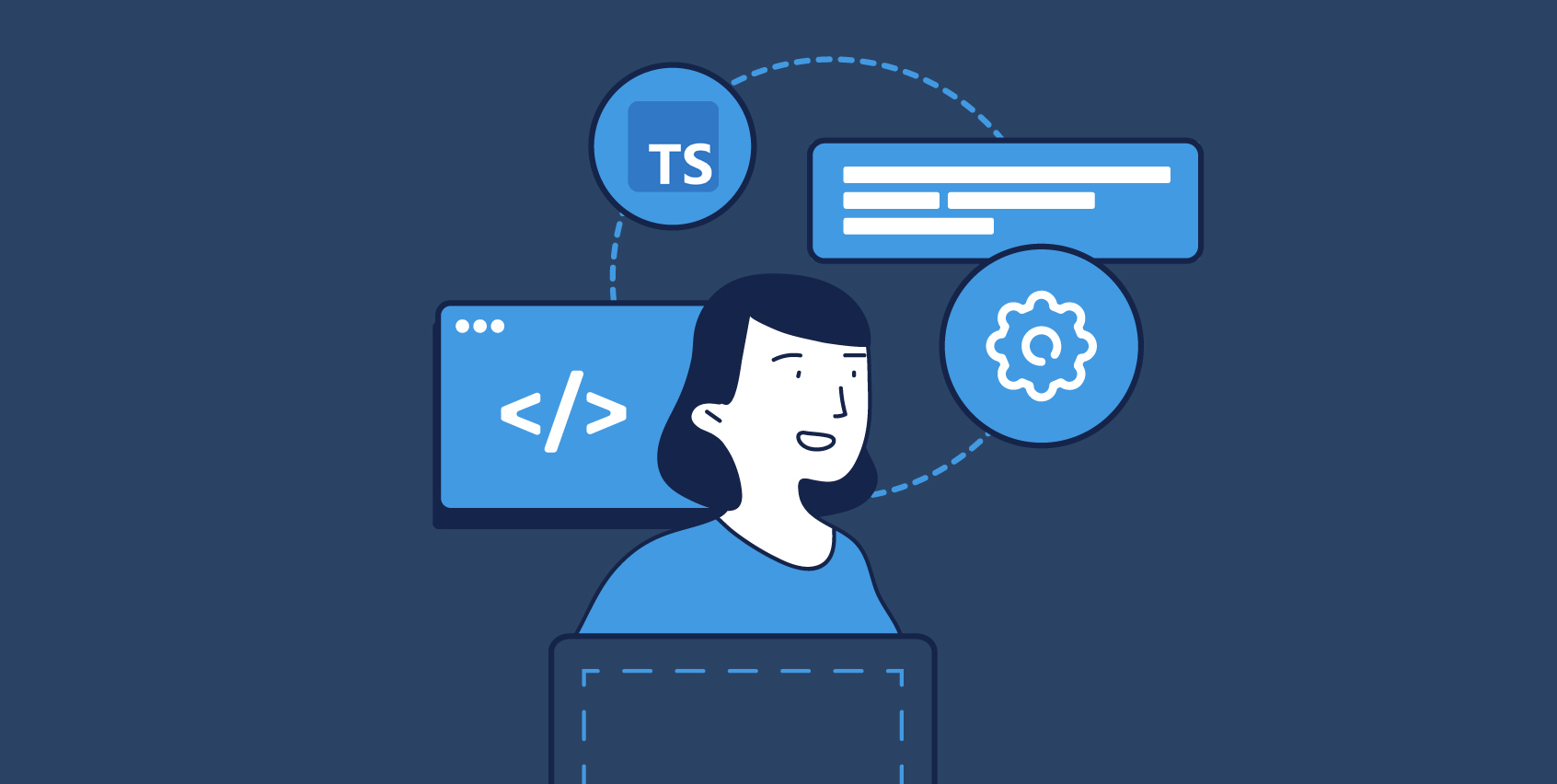
Web browsers have been quite administered for the past 10+ years, with JavaScript emerging along the way, to be used on servers and other devices. Websites are made up of HTML / XML documents, wherein these documents are static and do not change. TypeScript, being the type-based superset of JavaScript, makes the type definitions to the documented websites being readily available in any TypeScript project. This is carried out by Document Object Model (DOM) API where these definitions are placed in lib.dom.d.ts
. You can explore them on https://github.com/microsoft/TypeScript/blob/main/lib/lib.dom.d.ts.
Before we dive into how DOM manipulation is implemented in TypeScript, let us first learn what DOM is and how it is seen in web browsers. According to MDN Web Documentation:
"The Document Object Model (DOM) is a programming interface for HTML and XML documents. It represents the page so that programs can change the document structure, style, and content. The DOM represents the document as nodes and objects. That way, programming languages can connect to the page."
As we have learned from the above definition that how DOM is used to change the document structure, The API associated with it is so robust that frontend frameworks such as React, Angular, jQuery make dynamic websites much easier to build as these frameworks are developed around it.
Manipulating DOM (Implementation)
Considering a basic example as below:
Let us now explore some TypeScript code in our app.ts
file which inputs the name and age of a person and then greets them with a hello message, depicting the name and age on the webpage. It is very important to note here that this functionality is carried out using HTMLElement
which is the backbone definition for DOM manipulation. This is represented in the HTML document tree and is the base type for all other HTML elements such as div, span, image, etc.
const inputName = document.querySelector('#name') as HTMLInputElement;
const inputAge = document.querySelector('#age') as HTMLInputElement;
const inputForm = document.querySelector('form')!;
const hello = document.querySelector('hello') as HTMLDivElement;
interface PersonInterface {
name: string;
age: number;
}
class Person implements PersonInterface {
constructor (public name: string, public age: number) {}
hello() {
return `Hello, my name is ${this.name}, I am ${this.age} years old.`;
}
}
inputForm.addEventListener('submit', (e) => {
e.preventDefault();
const person = new Person(inputName.value, inputAge.valueAsNumber);
hello.innerText = person.hello();
inputForm.reset();
})
The above code snippet shows how querySelector() is used to read the name, age, form, greet inputs and then storing them in their respective HTML input elements. This method returns the first element which is present within the document, matching the selector of that particular element. If no matches are found, then null
is returned.
Further diving more into the code, we have defined a constructor function as used typically in our TypeScript code, taking name and age parameters. The hello() function then gets implemented by returning the greeting message with name and age. Using the innerText property of DOM, we want our message to be displayed in a div element after name and age get submitted into the form. Using reset property makes the form render from the beginning into our input form element if we want to test it further by adding the name and age of a different person.
Conclusion
The above implementation revises the following pointers:
HTMLElement
to be used when we want to work with form input elements.querySelector()
method is used both with the Document and Element interface and gets called when their group of selectors for the input element gets a match.
Leave a reaction to my article if you like it!
Thanks for reading!